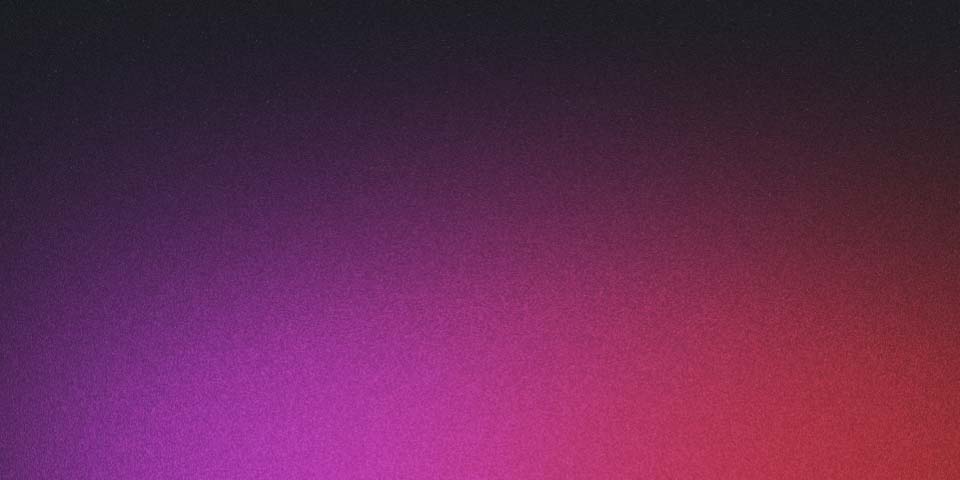
Making your own programming language in 5 minutes
what the title says, duh
Making your own programming language in 5 minutes
ok no BS let’s cut to the chase
Programming languages are just a bunch of rules that tell a programer how to write code to make computer do stuff. So let’s make our own language that does something simple.
it will be called “Bitch” and it will only have one command: Yell
which will print whatever comes after it to the console.
we will do it the under engineering way. We will write the simplest compiler that works. It won’t be very good, but it will be good learning experience, and help us understand the problem better. then we can make it better.
Here’s how you can write a program in Bitch:
Yell "Hello, World!"
and here what the computer needs to see:
segment .data
msg db "Hello, World!", 0
segment .text
global _start
_start:
mov eax, 4
mov ebx, 1
mov ecx, msg
mov edx, 13
int 0x80
mov eax, 1
mov ebx, 0
int 0x80
see how much they differ? Just like you and your tinder pics. that’s what the compiler does. It takes the simple language that you wrote and converts it to something that the computer can understand. Garbage in, garbage out.
”technically” this is assembly code, but it’s close enough to machine code that we can just write it directly to a file and run it( assuming a bunch of stuff that we’re not going to worry about right now ).
So yeah compiler is just a program that takes one text and converts it to another text. It’s like a translator but for computers.
oke so let’s write a compiler for Bitch. We’re going to write it in python because it’s easy to read and write. Here’s the code, don’t forget the very important and unhelpful comment that explain what the code does:
// this function takes a program written in Bitch and converts it to assembly code. log it to the console or write it to a file. or whatever.
function compile(program):
return program.replace("Yell", "segment .data\n msg db ").replace("!", ", 0\nsegment .text\n global _start\n_start:\n mov eax, 4\n mov ebx, 1\n mov ecx, msg\n mov edx, 13\n int 0x80\n mov eax, 1\n mov ebx, 0\n int 0x80")
That’s it. That’s the whole compiler. It’s not very good, but it works for our simple language. You can run the assembled code here https://onecompiler.com/assembly/3w22x43ev
*optional:
ok so technically we’re not done yet. We need to make a .exe file that the computer can run. But that’s easy. Just write the assembled code to a file and run few commands. We can use NASM to assemble the code and LD to link it. Here’s how you can do it:
first you need to install NASM and LD. You can do that by running sudo apt-get install nasm
and sudo apt-get install ld
on linux. on windows/mac you can download them from the internet. https://www.nasm.us/ and https://www.gnu.org/software/binutils/
then you can run the following commands:
nasm -f elf32 program.asm
ld -m elf_i386 -s -o program program.o
./program
program.asm is the file that contains the assembled code from the compiler we wrote. program is the file that the computer can run. you can name them whatever you want.
and that’s it. you just wrote a compiler in 5 minutes. congratulations. you’re now a programmer. go get a job at google or something.
Okay now for the real talk. This is a very simple compiler. It only works for one command and it doesn’t do any error checking. But it’s a good starting point. Real compilers are much more complicated and consists of many different parts ( more on that in next posts ). They have to deal with a lot of different commands and they have to be able to handle errors gracefully. But the basic idea is the same. They take a program written in a high-level language and convert it to a low-level language that the computer can understand.
If you’re interested in learning more about compilers, tinny tiny compiler is a good place to start.
I hope this was helpful. If you have any questions, feel free to ask. I’m always happy to help. Good luck with your programming adventures! :)